Java基础第五讲:流程控制(一)
如果您发现本文排版有问题,可以先点击下面的链接切换至老版进行查看!!!
Java基础第五讲:流程控制(一)

public class Lesson06_1 { public static void main(String[] args) { //设置黄文强在 boolean flag = true; System.out.println("开始"); if (flag){ System.out.println("在"); } System.out.println("结束"); } }为了把分支语句的前后界定清楚,我加了开始和结束标识。上面的运行结果是:
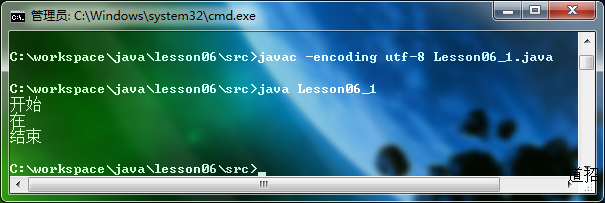

public class Lesson06_1 { public static void main(String[] args) { //设置黄文强不在 boolean flag = false; System.out.println("开始"); if (flag){ System.out.println("在"); }else{ System.out.println("他不在"); } System.out.println("结束"); } }上面的运行结果是:
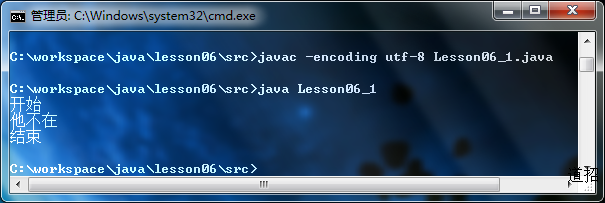
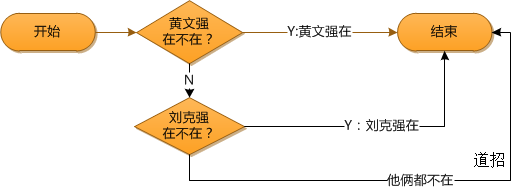
public class Lesson06_1 { public static void main(String[] args) { // 设置黄文强不在 boolean flag1 = false; // 设置刘克强在 boolean flag2 = true; System.out.println("开始->"); if (flag1) { System.out.println("黄文强在"); } else if (flag2) { System.out.println("刘克强在"); } System.out.println("->结束"); } }上面的运行结果是:
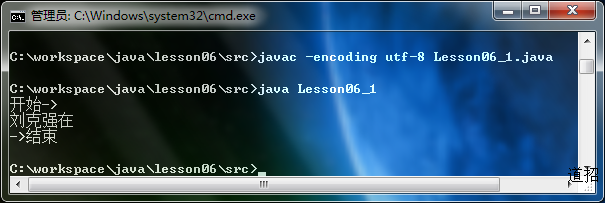
public class Lesson06_1 { public static void main(String[] args) { // 设置黄文强不在 boolean flag1 = false; // 设置刘克强在 boolean flag2 = true; System.out.println("开始->"); if (flag1) { System.out.println("黄文强在"); } else if (flag2) { System.out.println("刘克强在"); } else { System.out.println("他们不在"); } System.out.println("->结束"); } }上面的运行结果是:
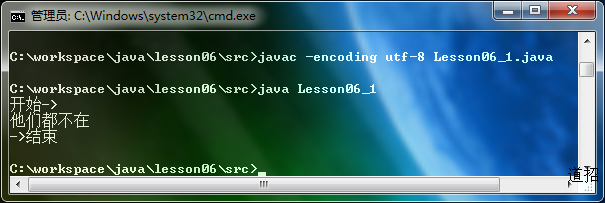
- if后的括号不能省略,括号里表达式的值最终必须返回的是布尔值
- 如果条件体内只有一条语句需要执行,那么if后面的大括号可以省略,但这是一种极为不好的编程习惯。
- 对于给定的if,else语句是可选的,else if 语句也是可选的
- else和else if同时出现时,else必须出现在else if 之后
- 如果有多条else if语句同时出现,那么如果有一条else if语句的表达式测试成功,那么会忽略掉其他所有else if和else分支。
- 如果出现多个if,只有一个else的情形,else子句归属于最内层的if语句
public class Lesson06 { public static void main(String[] args) { boolean examIsDone = true; int score = 65; if (examIsDone) if (score >= 90)System.out.println("A ,Excellent"); else if (score >= 80) System.out.println("B ,Good"); else if (score >= 70) System.out.println("C ,Middle"); else if (score >= 60) System.out.println("D ,Pass"); else System.out.println("E ,Fail"); System.out.println("Done is Done"); } }你认为else是属于哪个if语句的?System.out.println(“Done is Done”);是在哪一行代码之后执行的? 二、分支控制语句 switch Statement Java中有一个和if语句比较相似的分支控制语句叫switch ,它在有一系列固定值做分支时使用效率要比if-else方式效率高(别急,等一下再告诉你为什么效率高)。 先看一个例子:假设我们不考虑闰年的话,我们如何知道一个月有多少天?先用if-else的方式来实现:
public class Lesson06_2 { public static void main(String[] args) { int month=9; if(month==1){ System.out.println(month+"月有31天"); }else if(month==2){ System.out.println(month+"月有28天"); }else if(month==3){ System.out.println(month+"月有31天"); }else if(month==4){ System.out.println(month+"月有30天"); }else if(month==5){ System.out.println(month+"月有31天"); }else if(month==6){ System.out.println(month+"月有30天"); }else if(month==7){ System.out.println(month+"月有31天"); }else if(month==8){ System.out.println(month+"月有31天"); }else if(month==9){ System.out.println(month+"月有30天"); }else if(month==10){ System.out.println(month+"月有31天"); }else if(month==11){ System.out.println(month+"月有30天"); }else if(month==12){ System.out.println(month+"月有31天"); }else{ System.out.println("没有这个月份吧"); } } }接下来我们使用switch语句重新实现一次:
public class Lesson06_4 { public static void main(String[] args) { int month = 9; switch (month) { case 1: System.out.println(month + "月有31天"); break; case 2: System.out.println(month + "月有28天"); break; case 3: System.out.println(month + "月有31天"); break; case 4: System.out.println(month + "月有30天"); break; case 5: System.out.println(month + "月有31天"); break; case 6: System.out.println(month + "月有30天"); break; case 7: System.out.println(month + "月有31天"); break; case 8: System.out.println(month + "月有31天"); break; case 9: System.out.println(month + "月有30天"); break; case 10: System.out.println(month + "月有31天"); break; case 11: System.out.println(month + "月有30天"); break; case 12: System.out.println(month + "月有31天"); break; default: System.out.println("没有这个月份吧"); break; } } }运行2个程序,结果都是 9月有30天。
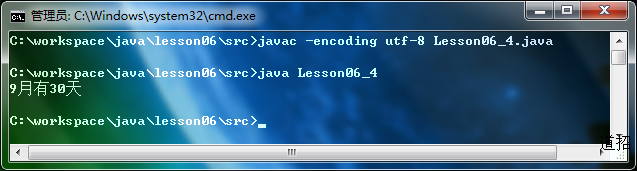
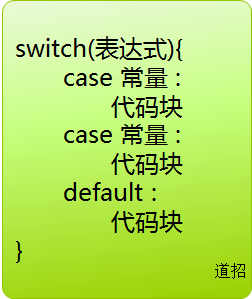
public class Lesson06_5 { public static void main(String[] args) { final int a = 2; final int b; b = 3; int x =2; switch(x){ case 1: //编译OK case a: //编译OK case b: //无法通过编译 } } }编译一下看看:
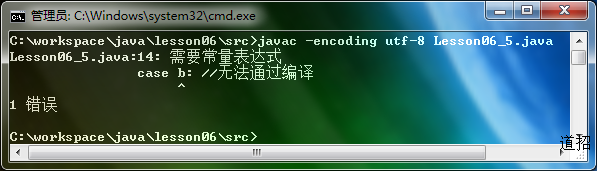
public class Lesson06_5 { public static void main(String[] args) { byte x =2; switch(x){ case 1: //编译OK case 128: //无法自动转换成byte,编译器会报错 } } }
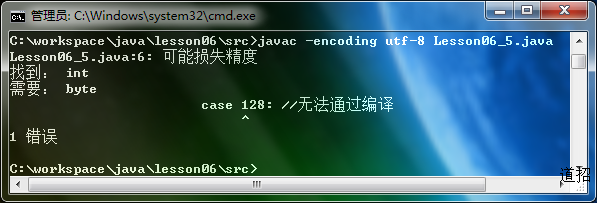
public class Lesson06_5 { public static void main(String[] args) { byte x =2; switch(x){ case 1: //编译OK case 1: } } }编译一下看看:
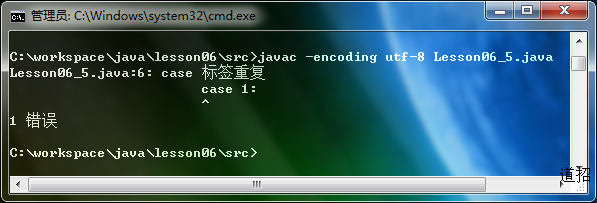
public class Lesson06_5 { public static void main(String[] args) { int x = 1; switch (x) { case 1:System.out.println("周一"); case 2:System.out.println("周二"); case 3:System.out.println("周三"); case 4:System.out.println("周四"); case 5:System.out.println("周五"); case 6:System.out.println("周六"); case 7:System.out.println("周日"); default:System.out.println("这个是星期几啊"); } } }
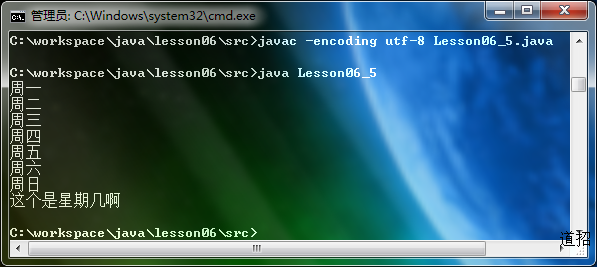
public class Lesson06_3 { public static void main(String[] args) { int month = 9; switch (month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: System.out.println(month + "月有31天"); break; case 2: System.out.println(month + "月有28天"); break; case 4: case 6: case 9: case 11: System.out.println(month + "月有30天"); break; default: System.out.println("没有这个月份吧"); break; } } }运行程序:
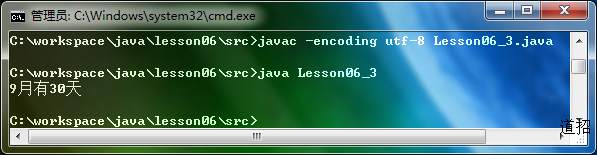
public class Lesson06_5 { public static void main(String[] args) { int x = 8; switch (x) { case 1:System.out.println("周一"); default:System.out.println("这个是星期几啊"); case 2:System.out.println("周二"); case 3:System.out.println("周三"); case 4:System.out.println("周四"); case 5:System.out.println("周五"); break; case 6:System.out.println("周六"); case 7:System.out.println("周日"); } } }运行程序:
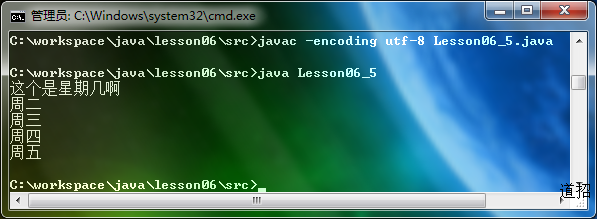
- 分类:
- Java
更新时间:
相关文章
Java基础第六讲:流程控制(二)
本讲内容:循环、跳出循环、标签跳转 Java中循环有三种形式 while循环、do-while循环 和 for循环。其中从Java 6 开始for循环又分 普通for循环 和 for-each循 阅读更多…